Setup a simple CI/CD Pipeline with Jenkins
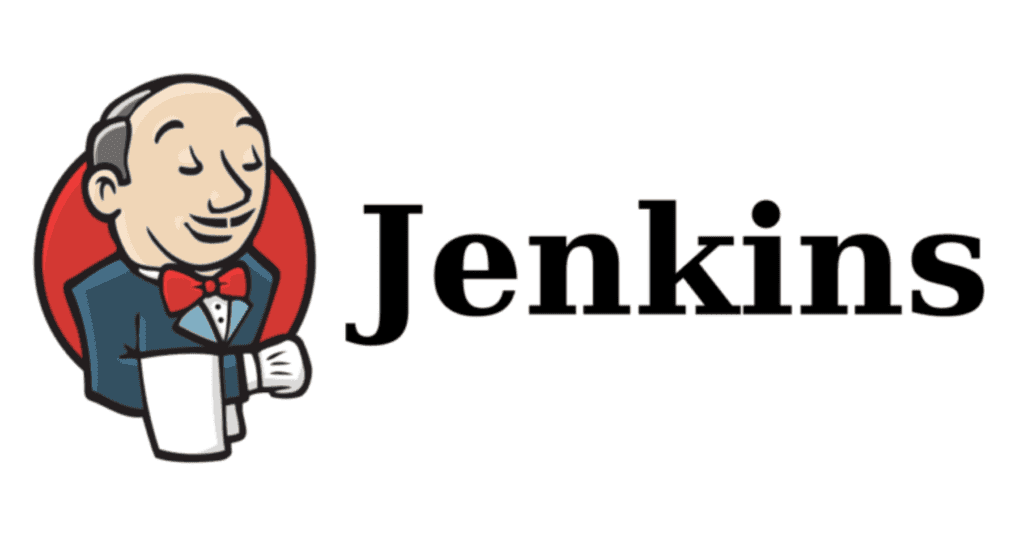
Prerequisites
Before we begin, make sure you have:
✅ Jenkins installed (Locally or on a server)
✅ Git installed and a repository to use
✅ A basic project (e.g., a simple Node.js or Python app)
✅ Java installed (Jenkins runs on Java)
Step 1: Install Required Jenkins Plugins
- Open Jenkins Dashboard → Manage Jenkins → Manage Plugins
- Under Available Plugins, install the following plugins:
- Pipeline (For writing Jenkinsfile-based pipelines)
- Git (For Git repository integration)
- SSH Pipeline Steps (For SSH-based deployment)
- Restart Jenkins after installing the plugins.
Step 2: Create a New Jenkins Job
- Go to the Jenkins Dashboard → Click New Item
- Select Pipeline and give it a meaningful name (e.g., "My CI/CD Pipeline")
- Click OK
Step 3: Connect Jenkins to Your Git Repository
- Inside your new job, go to Configure
- Under Pipeline → Definition, select Pipeline script from SCM
- Under SCM, choose Git, and enter your repository URL
- If the repo is private, add credentials
- Set the branch (e.g., main or develop)
Step 4: Create a Jenkinsfile for Your Pipeline
In your project root, create a file named Jenkinsfile and add:
pipeline {
agent any
stages {
stage('Clone Repository') {
steps {
git 'https://github.com/your-repo.git'
}
}
stage('Build') {
steps {
sh 'echo "Building the project..."'
}
}
stage('Test') {
steps {
sh 'echo "Running tests..."'
}
}
stage('Deploy') {
steps {
sh 'echo "Deploying application..."'
}
}
}
}
Step 5: Run Your Pipeline
- Go back to Jenkins Dashboard → Select your pipeline
- Click Build Now
- Watch your pipeline execute in Build History
- Check the console output for any errors.
- If successful, you'll see a green checkmark.
Bonus: Automate with Webhooks
To trigger the pipeline automatically on every commit:
- In GitHub/GitLab, go to Repository Settings → Webhooks
- Add your Jenkins URL:
http://your-jenkins-url/github-webhook/
- Select Push Events to trigger on new commits.
Now, Jenkins will run the pipeline whenever you push code!